Tuesday, December 29, 2009
How to merge tables in a Dataset - Sql Server
The DataSet contains copy of the data we requested through the SQL statement. The SqlDataAdapter object allows us to populate DataTable in a DataSet. We can use Fill method in the SqlDataAdapter for populating data in a Dataset. We can populate Dataset with more than one table at a time using SqlDataAdapter Object. The DataTableCollection contains zero or more DataTable objects.
In some situation we want to combine the result of multiple SQL query as a single result set. In that case we can use the Dataset's Merge method for doing this. The tables involved in the merge should be identical, that is the columns are similar data types .
Private Sub GetUsersList()
Dim connetionString As String
Dim connection As SqlConnection
Dim command As SqlCommand
Dim adapter As New SqlDataAdapter
Dim ds As New DataSet
Dim dt As DataTable
Dim firstSql As String
Dim secondSql As String
Dim i As Integer
connetionString = "Data Source=.;Initial Catalog=Nafex;User ID=sa;Password=111"
firstSql = "Select * from tblUser"
secondSql = "Select * from tluVrType"
connection = New SqlConnection(connetionString)
Try
connection.Open()
command = New SqlCommand(firstSql, connection)
adapter.SelectCommand = command
adapter.Fill(ds, "Table(0)")
adapter.SelectCommand.CommandText = secondSql
adapter.Fill(ds, "Table(1)")
adapter.Dispose()
command.Dispose()
connection.Close()
ds.Tables(0).Merge(ds.Tables(1))
dt = ds.Tables(0)
For i = 0 To dt.Rows.Count - 1
MsgBox(dt.Rows(i).Item(0) & " -- " & dt.Rows(i).Item(1))
Next
Catch ex As Exception
MsgBox("Can not open connection ! ")
End Try
End Sub
Sunday, December 20, 2009
String Format for Double ( 0.00)
The following examples show how to format float numbers to string in C#. You can use static method String.Format or instance methods double.ToString and float.ToString.
Digits after decimal point
This example formats double to string with fixed number of decimal places. For two decimal places use pattern „0.00“. If a float number has less decimal places, the rest digits on the right will be zeroes. If it has more decimal places, the number will be rounded.
[C#]// just two decimal places
String.Format("{0:0.00}", 123.4567); // "123.46"
String.Format("{0:0.00}", 123.4); // "123.40"
String.Format("{0:0.00}", 123.0); // "123.00"
Next example formats double to string with floating number of decimal places. E.g. for maximal two decimal places use pattern „0.##“.
[C#]// max. two decimal places
String.Format("{0:0.##}", 123.4567); // "123.46"
String.Format("{0:0.##}", 123.4); // "123.4"
String.Format("{0:0.##}", 123.0); // "123"
Digits before decimal point
If you want a float number to have any minimal number of digits before decimal point use N-times zero before decimal point. E.g. pattern „00.0“ formats a float number to string with at least two digits before decimal point and one digit after that.
[C#]// at least two digits before decimal point
String.Format("{0:00.0}", 123.4567); // "123.5"
String.Format("{0:00.0}", 23.4567); // "23.5"
String.Format("{0:00.0}", 3.4567); // "03.5"
String.Format("{0:00.0}", -3.4567); // "-03.5"
Thousands separator
To format double to string with use of thousands separator use zero and comma separator before an usual float formatting pattern, e.g. pattern „0,0.0“ formats the number to use thousands separators and to have one decimal place.
[C#]String.Format("{0:0,0.0}", 12345.67); // "12,345.7"
String.Format("{0:0,0}", 12345.67); // "12,346"
Zero
Float numbers between zero and one can be formatted in two ways, with or without leading zero before decimal point. To format number without a leading zero use # before point. For example „#.0“ formats number to have one decimal place and zero to N digits before decimal point (e.g. „.5“ or „123.5“).
Following code shows how can be formatted a zero (of double type).
[C#]String.Format("{0:0.0}", 0.0); // "0.0"
String.Format("{0:0.#}", 0.0); // "0"
String.Format("{0:#.0}", 0.0); // ".0"
String.Format("{0:#.#}", 0.0); // ""
Align numbers with spaces
To align float number to the right use comma „,“ option before the colon. Type comma followed by a number of spaces, e.g. „0,10:0.0“ (this can be used only in String.Format method, not in double.ToString method). To align numbers to the left use negative number of spaces.
[C#]String.Format("{0,10:0.0}", 123.4567); // " 123.5"
String.Format("{0,-10:0.0}", 123.4567); // "123.5 "
String.Format("{0,10:0.0}", -123.4567); // " -123.5"
String.Format("{0,-10:0.0}", -123.4567); // "-123.5 "
Custom formatting for negative numbers and zero
If you need to use custom format for negative float numbers or zero, use semicolon separator „;“ to split pattern to three sections. The first section formats positive numbers, the second section formats negative numbers and the third section formats zero. If you omit the last section, zero will be formatted using the first section.
[C#]String.Format("{0:0.00;minus 0.00;zero}", 123.4567); // "123.46"
String.Format("{0:0.00;minus 0.00;zero}", -123.4567); // "minus 123.46"
String.Format("{0:0.00;minus 0.00;zero}", 0.0); // "zero"
Some funny examples
As you could notice in the previous example, you can put any text into formatting pattern, e.g. before an usual pattern „my text 0.0“. You can even put any text between the zeroes, e.g. „0aaa.bbb0“.
[C#]String.Format("{0:my number is 0.0}", 12.3); // "my number is 12.3"
String.Format("{0:0aaa.bbb0}", 12.3); // "12aaa.bbb3"
Wednesday, December 09, 2009
OFAC Block Person List
The Office of Foreign Assets Control ("OFAC") of the US Department of the Treasury administers and enforces economic and trade sanctions based on US foreign policy and national security goals against targeted foreign countries and regimes, terrorists, international narcotics traffickers, those engaged in activities related to the proliferation of weapons of mass destruction, and other threats to the national security, foreign policy or economy of the United States. OFAC acts under Presidential national emergency powers, as well as authority granted by specific legislation, to impose controls on transactions and freeze assets under US jurisdiction. Many of the sanctions are based on United Nations and other international mandates, are multilateral in scope, and involve close cooperation with allied governments.
http://www.treas.gov/offices/enforcement/ofac/sdn/index.shtml
OFAC web services into our project (OFAC with .NET VB/V#)
There are many web services online live sites who provided the OFAC web services.
But this OFAC web services is not free only for Free try Testing after that Buy the OFAC web services and implement into project.
I Implement one of the OFAC web services here are the Steps for Implementation OFAC.
this is one of the best site for OFAC web services i m useing this .
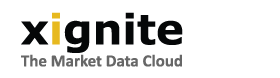
https://www.xignite.com/Login.aspx?ReturnUrl=/MyAccount/MyAccountHome.aspx&mesg=yes
Step 1:
Create Registration
Step 1:
Login into your Account.
Step 3:
Create VB.NET Project (Web Project)
Step 4:
Header Code for OFAC Web services Code into your Project.
Dim objSearchResults As New com.xignite.www.SearchResults
'RemoteQuotes is the web reference to the XigniteQuotes service
'objRemoteQuote is an instance of the service proxy
Dim objRemoteQuote As New com.xignite.www.Header
'assign your username / password , same as you Registration on xignite.com
objRemoteQuote.Password = "*******
objRemoteQuote.Username = "*****@****.com"
'assign the header to the service proxy
objOFACService.HeaderValue = objRemoteQuote
'----------------------------------------Searching OFAC CODE
objOFACService.SearchByAddressAsync(com.xignite.www.OFACTypes.All , "Person Name","Address" , "City" ,"Country" , com.xignite.www.SearchTypes.Contains )
Dim ct, adct As Integer
For ct = 0 To result.Count
address = result.Matches(ct).Addresses.ToString()
If result.Matches(ct).Addresses.Length > 0 Then
For adct = 0 To result.Matches(ct).Addresses.Length
txtaddress.Text = result.Matches(ct).Addresses(adct).Country.ToString()
OfacChk = True
OfacResponsIDnumb = result.Matches(ct).Id
Next
End If
Next
========================================
OFAC Person match in Live OFAC List.
by Asma Qureshi
Project manager
Dubai UAE