Introduction:
In this article I will explain how to implement URL Routing in ASP.Net Web Forms 4.0.
URL Routing is supported in .Net 3.5 SP1 or higher frameworks, if you want to use it in Visual Studio 2008 you will need to install .Net Framework 3.5 Service Pack 1.
Adding Global.asax file
The first thing you need to do is add Global.asax file to the ASP.Net Website project using Add New Item dialog in Visual Studio.
For the purpose of illustrating the URL Routing in this article, I have added some ASP.Net pages to the project as show below.
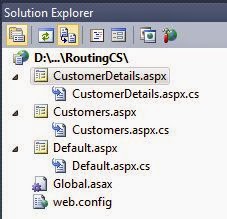
Simple Routing: Hide (Remove) .ASPX extension in URL in ASP.Net using URL Routing:
In order to implement URL Routing we will need to register the routes that we need to use in our application.
In order to do that I have created a method named RegisterRoutes and it has been called inside the Application_Start event inside the Global.asax file. This method will allow us to add routes to handle so that we can create our own custom URLs.
Application_Start event inside the Global.asax file:
Passing parameters to another page using URL Routing
In order to explain passing of parameters to from one page to another when using URL Routing, I will extend the same example by adding a GridView with some sample data to the Customers.aspx page and adding route for the page CustomerDetails.aspx.
Binding of GridView using sample data using DataTable has already been covered extensively in my article Dynamically create DataTable and bind to GridView in ASP.Net and hence I’ll not be covering it here.
The same GridView will be used here with an additional column containing a HyperLinkField which will be used to redirect to the CustomerDetails.aspx page along with the Customer ID of the corresponding record.
In this article I will explain how to implement URL Routing in ASP.Net Web Forms 4.0.
URL Routing is supported in .Net 3.5 SP1 or higher frameworks, if you want to use it in Visual Studio 2008 you will need to install .Net Framework 3.5 Service Pack 1.
Adding Global.asax file
The first thing you need to do is add Global.asax file to the ASP.Net Website project using Add New Item dialog in Visual Studio.
For the purpose of illustrating the URL Routing in this article, I have added some ASP.Net pages to the project as show below.
Simple Routing: Hide (Remove) .ASPX extension in URL in ASP.Net using URL Routing:
In order to implement URL Routing we will need to register the routes that we need to use in our application.
In order to do that I have created a method named RegisterRoutes and it has been called inside the Application_Start event inside the Global.asax file. This method will allow us to add routes to handle so that we can create our own custom URLs.
Application_Start event inside the Global.asax file:
<%@ Application Language="C#" %>
<%@ Import Namespace="System.Web.Routing" %>
<script runat="server">
void Application_Start(object sender, EventArgs e)
{
RegisterRoutes(RouteTable.Routes);
}
static void RegisterRoutes(RouteCollection routes)
{
routes.MapPageRoute("Customers", "Customers", "~/Customers.aspx");
}
</script>
Inside the RegisterRoutes method, I have made use of MapPageRoute method which accepts the following three parameters.
1. routeName: Name of the Route. Must be unique for each route. You can set any unique name, I have named it as Customers for better understanding.
2. routeUrl: The Route URL you want to implement. For example here we want Customers.aspx to appear as only Customers (without .ASPX extension), thus this customization has to be defined here.
3. physicalFile: The URL of the actual ASP.Net Page where the Route URL should redirect to. For this example it is Customers.aspx.
The MapPageRoute method adds the Route to the Global Route collection i.e. RouteTable.Routes.
The RegisterRoutes is called inside the Application_Start event handler so that all the routes are added the RouteTable collection when the application starts.
That’s all you need to do the remove or hide the .ASPX extension of the ASP.Net Web Page, now if you type in the URL as http://localhost:1932/RoutingCS/Customers/ or http://localhost:1932/RoutingCS/Customers both will redirect you to the customers page i.e. Customers.aspx.
1. routeName: Name of the Route. Must be unique for each route. You can set any unique name, I have named it as Customers for better understanding.
2. routeUrl: The Route URL you want to implement. For example here we want Customers.aspx to appear as only Customers (without .ASPX extension), thus this customization has to be defined here.
3. physicalFile: The URL of the actual ASP.Net Page where the Route URL should redirect to. For this example it is Customers.aspx.
The MapPageRoute method adds the Route to the Global Route collection i.e. RouteTable.Routes.
The RegisterRoutes is called inside the Application_Start event handler so that all the routes are added the RouteTable collection when the application starts.
That’s all you need to do the remove or hide the .ASPX extension of the ASP.Net Web Page, now if you type in the URL as http://localhost:1932/RoutingCS/Customers/ or http://localhost:1932/RoutingCS/Customers both will redirect you to the customers page i.e. Customers.aspx.
Passing parameters to another page using URL Routing
In order to explain passing of parameters to from one page to another when using URL Routing, I will extend the same example by adding a GridView with some sample data to the Customers.aspx page and adding route for the page CustomerDetails.aspx.
Binding of GridView using sample data using DataTable has already been covered extensively in my article Dynamically create DataTable and bind to GridView in ASP.Net and hence I’ll not be covering it here.
The same GridView will be used here with an additional column containing a HyperLinkField which will be used to redirect to the CustomerDetails.aspx page along with the Customer ID of the corresponding record.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Id" HeaderText="Id" ItemStyle-Width="30" />
<asp:BoundField DataField="Name" HeaderText="Name" ItemStyle-Width="150" />
<asp:BoundField DataField="Country" HeaderText="Country" ItemStyle-Width="150" />
<asp:HyperLinkField Text = "View" DataNavigateUrlFormatString = "~/Customers/{0}" DataNavigateUrlFields = "Id" />
</Columns>
</asp:GridView>
<%@ Application Language="C#" %>
<%@ Import Namespace="System.Web.Routing" %>
<script runat="server">
void Application_Start(object sender, EventArgs e)
{
RegisterRoutes(RouteTable.Routes);
}
static void RegisterRoutes(RouteCollection routes)
{
routes.MapPageRoute("Customers", "Customers", "~/Customers.aspx");
routes.MapPageRoute("CustomerDetails", "Customers/{CustomerId}", "~/CustomerDetails.aspx");
}
</script>
Above you will notice that there’s a new route added for CustomerDetails page just below the Customer’s page route.
But this route has a place holder {CustomerId} which will be replaced by actual Customer ID of the customer. Thus the URL to the CustomerDetails.aspx will now look like http://localhost:1932/RoutingCS/Customers/1 here the digit 1 is the ID of the Customer John Hammond.
Fetching parameters from URL passed from previous page using URL Routing
On the CustomerDetails page I have added a Label to display the Customer ID that was passed from the previous page.
But this route has a place holder {CustomerId} which will be replaced by actual Customer ID of the customer. Thus the URL to the CustomerDetails.aspx will now look like http://localhost:1932/RoutingCS/Customers/1 here the digit 1 is the ID of the Customer John Hammond.
Fetching parameters from URL passed from previous page using URL Routing
On the CustomerDetails page I have added a Label to display the Customer ID that was passed from the previous page.
<b>Customer Id:</b>
<asp:Label ID="lblCustomerId" runat="server" />
No comments:
Post a Comment